Google Fonts is an excellent resource for adding beautiful typography to your React project. When combined with Tailwind CSS, it allows you to maintain a clean, scalable, and utility-first approach to styling. In this guide, we’ll walk through the steps to integrate Google Fonts into a React app using Tailwind CSS.
Why Use Google Fonts with Tailwind?
Google Fonts provides a vast collection of free, high-quality fonts that can enhance the readability and aesthetics of your web application. Tailwind CSS, on the other hand, offers a utility-first approach to styling, making it easy to apply typography styles directly within your JSX.
Steps to Add Google Fonts to Your Tailwind React Project
1. Choose a Google Font
Visit Google Fonts and select a font you want to use. For example, let’s say you want to use Inter.
2. Add the Font to Your Project
There are two ways to add Google Fonts to your React project:
Option 1: Link in the HTML File (Recommended for Simplicity)
- Copy the
<link>
tag from Google Fonts. For Inter, it looks like this:<link href="https://fonts.googleapis.com/css2?family=Inter:wght@400;700&display=swap" rel="stylesheet">
- Open your
index.html
file (located inpublic/index.html
) and paste the link inside the<head>
tag<head> <link href="https://fonts.googleapis.com/css2?family=Inter:wght@400;700&display=swap" rel="stylesheet"> </head>
Option 2: Import via CSS (Recommended for Vite and Optimization)
If you’re using Vite, you can import the font in your index.css
or global.css
file:
@import url('https://fonts.googleapis.com/css2?family=Inter:wght@400;700&display=swap');
3. Extend Tailwind Configuration
To use the Google Font in Tailwind, add it below the import we done in previous step
@theme { --font-inter:'Inter', 'sans-serif' }
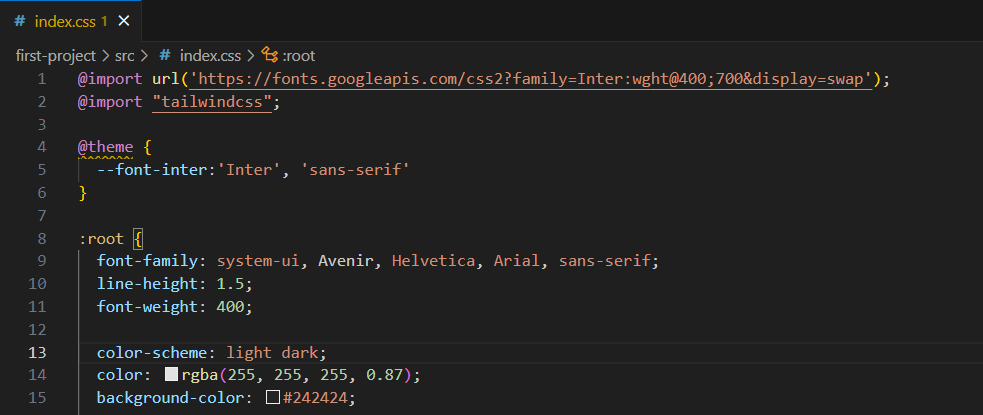
4. Apply the Font in Your Components
Now, you can use the font class in your React components:
function App() { return ( <div className="font-inter text-lg p-4"> <h1 className="text-3xl font-bold">Hello, Tailwind with Google Fonts!</h1> <p>This is a simple example of using Google Fonts in a Tailwind React app.</p> </div> ); } export default App;
5. Verify Your Changes
Run your React app and confirm that the font is applied correctly:
npm run dev # or yarn dev
If the font is not applied, check the following:
- Ensure the Google Font link or import statement is correctly added.
- Verify that Tailwind’s
fontFamily
configuration is correctly extended. - Clear cache and restart the dev server if needed.
Conclusion
Integrating Google Fonts into a React app with Tailwind CSS is straightforward. Whether you use a <link>
tag or import it via CSS, the combination of Tailwind’s utility-first approach and Google Fonts’ vast typography options can significantly enhance your web project’s design.
By following these steps, you can easily apply custom fonts and maintain a clean, scalable design system within your React application.