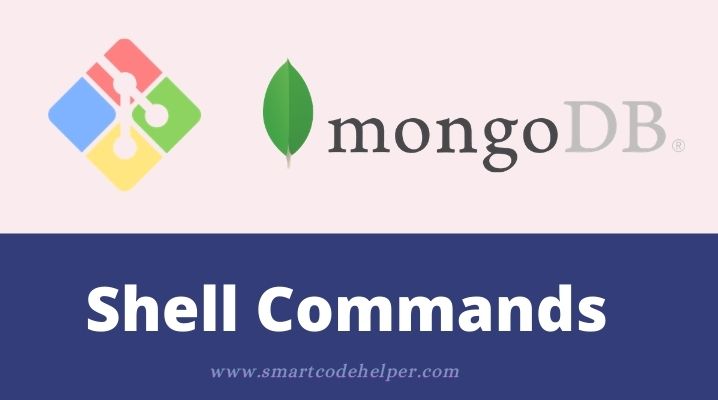
Intiate the db, by
mongod
Open new bash terminal and enter command
mongo
Now we can start using the mongo shell, just try the basic commands
help
Show Databases
show dbs
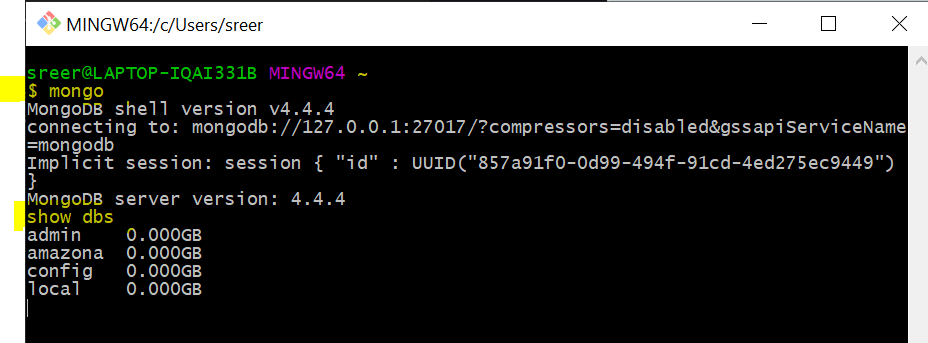
Create New Database
use newDBname
eg: use shopDB
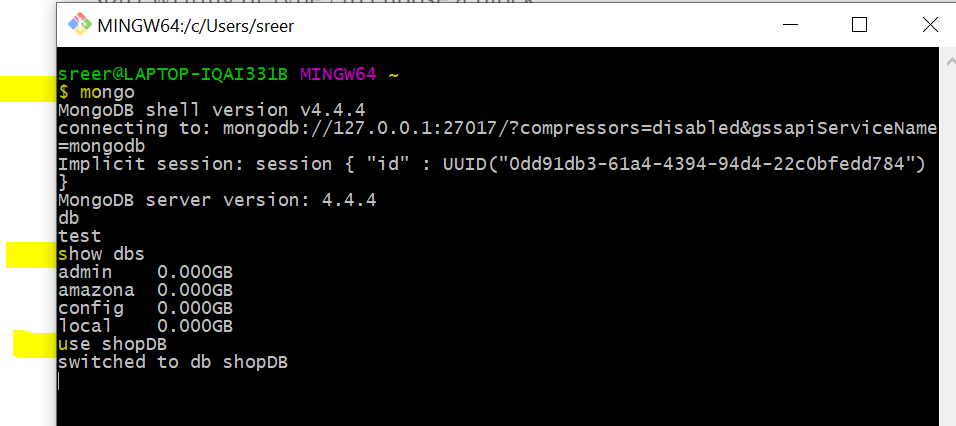
View Current Database
db
Create New Collection / Insert Data
Collections in Mongo DB is similar to tables in SQL. They are collection of document. Document is a single data record. In SQL its called a single row.
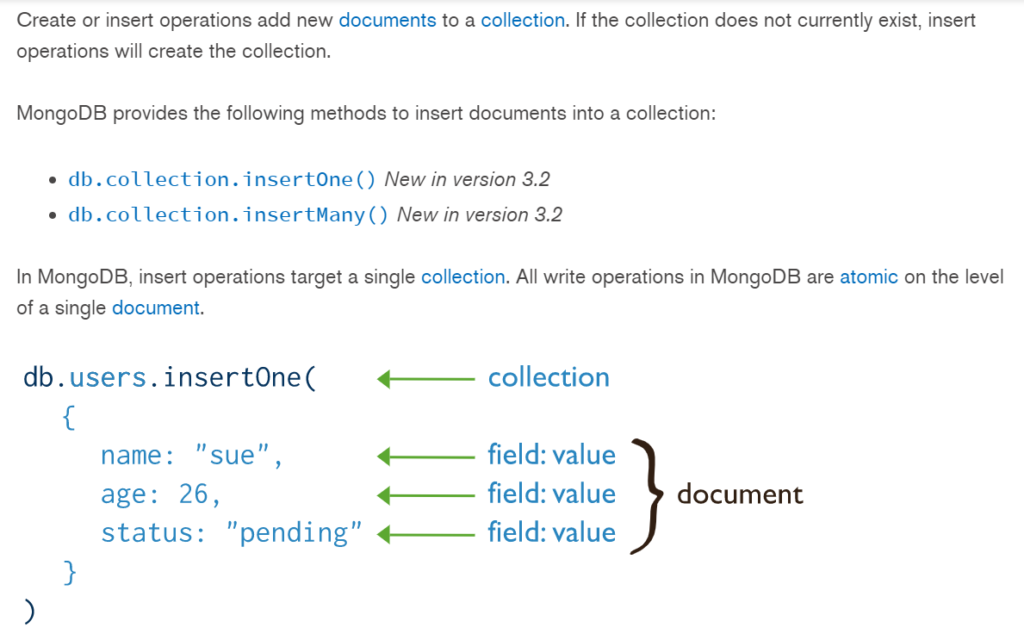
eg: db.products.insertOne({_id: 1, name: "Book", price:50})
View Collections
show collections
Read Data from Mongo Database
db.collection.find()
Eg: db.products.find()
Eg: db.products.find({name:"Book"})
Eg: db.products.find({price: {$gt: 10}})
Using Projections
Projects are used as the second parameter in the find(), it gets value 0 or 1
eg: db.products.find({_id:1}, {name:1, _id:0})
Update record in mongoDB
db.products.updateOne({_id: 1}, {$set: {stock:32}})
The first parameter is id and the second one is the new record.
Delete a record in mongoDB
db.products.deleteOne({_id: 2})
Relationships in mongoDB
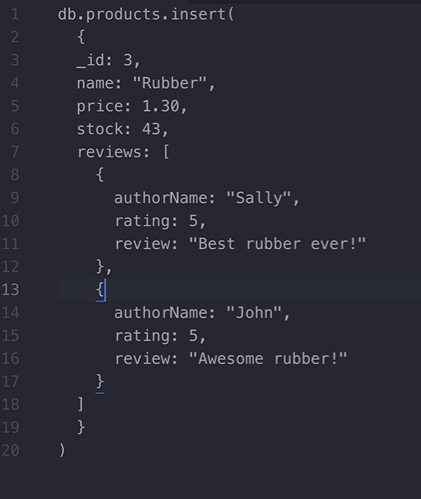
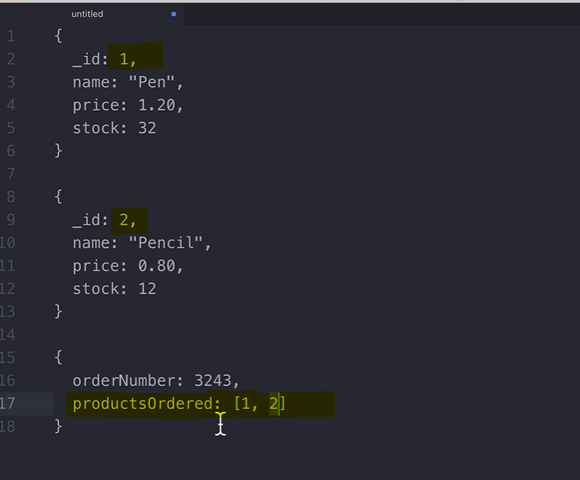
Delete a database in mongoDB
Firstly switch to the database,
use shopDB
Dropping the database
db.dropDatabase()