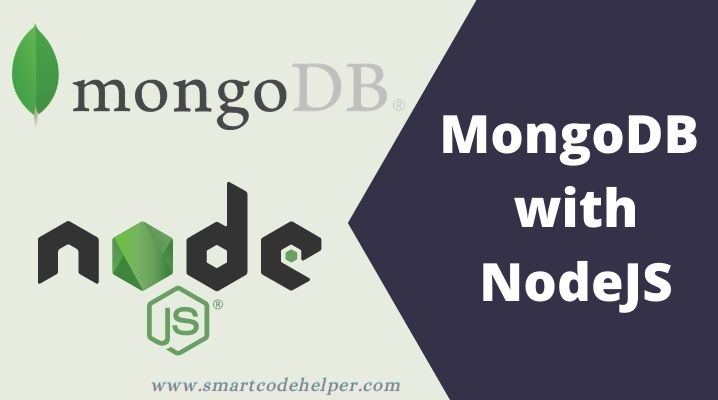
If we need to build Node JS apps using databases, then MongoDB is definitely a good option. MongoDB is a no-SQL database. For integrating MongoDB with a Node JS application we have two options.
One of the options is to use MongoDB native driver. The other option is by using ODM (Object Document Mapper) – Mongoose.
Most popular way of using MongoDB with Node JS is by using Mongoose. The main reason is because it vastly simplifies and reduces the code required to work with the MongoDB.
Using MongoDB native Driver
Refer: https://docs.mongodb.com/drivers/node/quick-start/
Using MongoDB with Mongoose
Setup Mongo DB in your system – Refer: Install and Setup MongoDB Database – Local
Then Setup the Node Project
Then we have to install the mongoose package
npm i mongoose
Example 1: Inserting single record in MongoDB using Node
Here we are creating a sample database called fruitsDB. Then we are trying to insert one record.
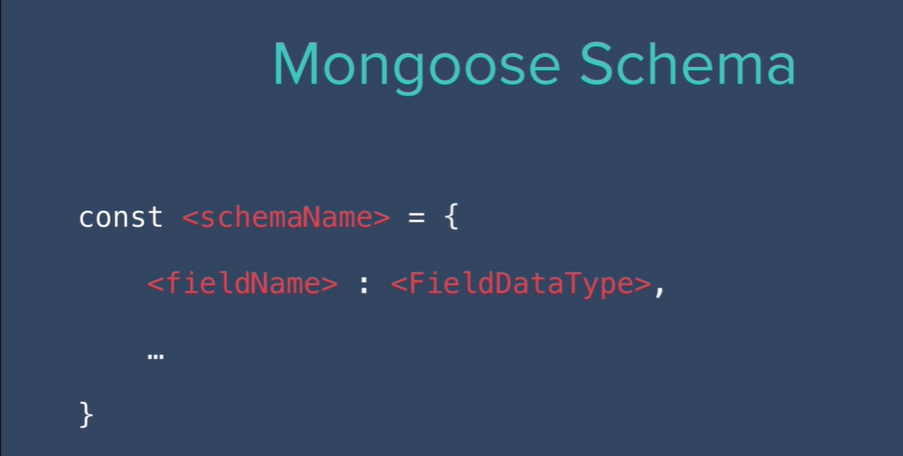
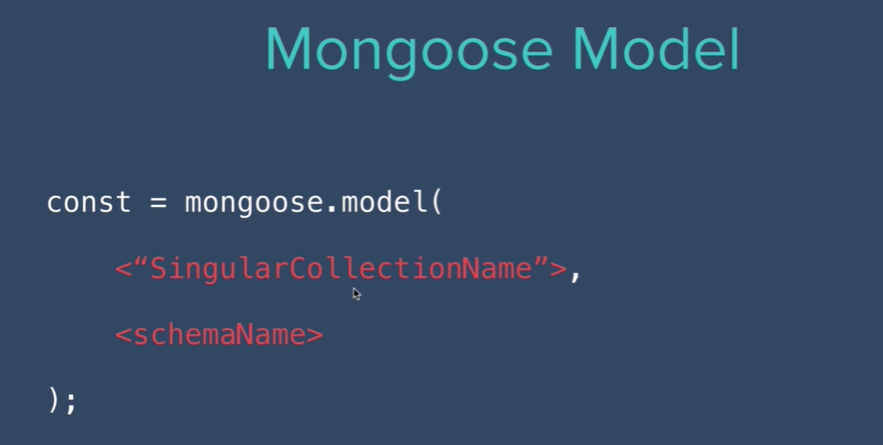
app.js
const mongoose = require('mongoose'); const uri = "mongodb://localhost:27017/fruitsDB" mongoose.connect(uri, {useNewUrlParser: true, useUnifiedTopology: true}); const fruitSchema = new mongoose.Schema ({ name: String, rating: Number, review: String }); const Fruit = mongoose.model("Fruit", fruitSchema); const fruit = new Fruit ({ name: "Apple", rating: 7, review: "Pretty solid as a fruit" }); person.save();
Example 2: Inserting multiple records in MongoDB using Node
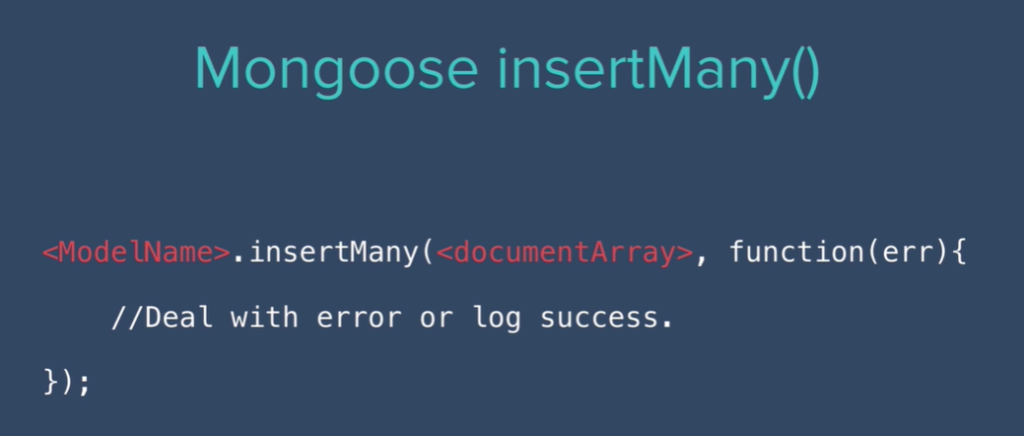
app.js
const mongoose = require('mongoose'); const uri = "mongodb://localhost:27017/fruitsDB" mongoose.connect(uri, {useNewUrlParser: true, useUnifiedTopology: true}); const fruitSchema = new mongoose.Schema ({ name: String, rating: Number, review: String }); const Fruit = mongoose.model("Fruit", fruitSchema); const orange = new Fruit({ name: "Orange", score: 4, review: "Too sour for me" }); const banana = new Fruit({ name: "Banana", score: 3, review: "Wierd texture" }); const kiwi = new Fruit({ name: "Kiwi", score: 4, review: "The best Fruit!" }); Fruit.insertMany([kiwi, orange, banana], function(err){ if(err){ console.log(err); } else{ console.log("Successfully saved all fruits to fruitsDB"); } });
Reading Data Records from MongoDB
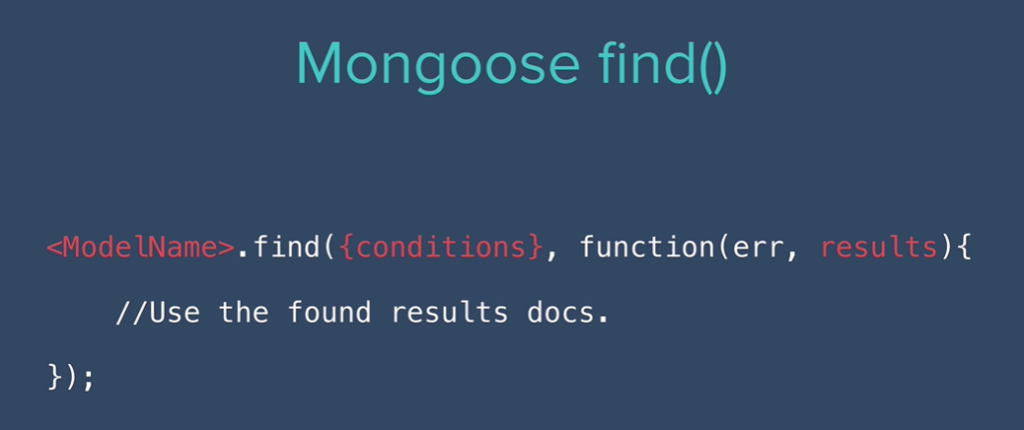
Fruit.find(function(err, fruits){ if(err){ console.log(err); } else{ console.log(fruits); } });
Accessing Property of MongoDB Record
Fruit.find(function(err, fruits){ if(err){ console.log(err); } else{ fruits.forEach(fruit => { console.log(fruit.name); }); } });
Closing database connection using mongoose
mongoose.connection.close();
For each for looping through and displaying data
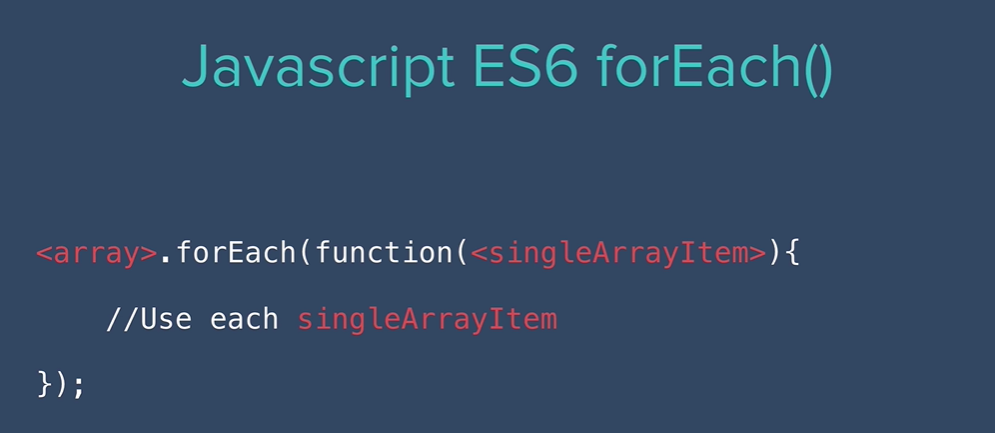