How to import JSON file data in React JS APP. Get data from json file and render inside react component example code.
data/items.json
[ { "id": 1, "name": "Book", "price": 10.99, "imgUrl": "/imgs/book.jpg" }, { "id": 2, "name": "Computer", "price": 1199, "imgUrl": "/imgs/computer.jpg" }, { "id": 3, "name": "Banana", "price": 1.05, "imgUrl": "/imgs/banana.jpg" }, { "id": 4, "name": "Car", "price": 14000, "imgUrl": "/imgs/car.jpg" } ]
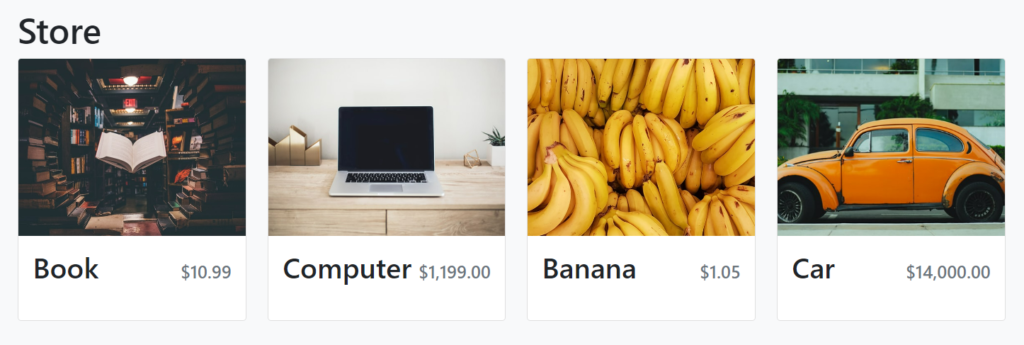
Importing and using json data in React App
Import JSON file data – React Example Code
import storeItems from "../data/items.json"
pages/Store.js
import { Col, Row } from "react-bootstrap"; import StoreItem from "../components/StoreItem"; import storeItems from "../data/items.json" const Store = () => { return <> <h1>Store</h1> <Row> { storeItems.map( item => ( <Col> <StoreItem {...item} /> </Col> )) } </Row> </>; }; export default Store;
components/StoreItem.tsx
import { Card } from "react-bootstrap" import { formatCurrency } from "../utilities/formatCurrency" type StoreItemProps = { id:number, name:string, price: number, imgUrl: string } const StoreItem = ({id, name, price, imgUrl} : StoreItemProps) => { return ( <Card> <Card.Img variant="top" src={imgUrl} height="200px" style={{ objectFit: "cover"}} /> <Card.Body className="d-flex flex-column"> <Card.Title className="d-flex justify-content-between align-items-baseline mb-4"> <span className="fs-2">{name}</span> <span className="ms-2 text-muted">{formatCurrency(price)}</span> </Card.Title> </Card.Body> </Card> ) } export default StoreItem