Fastify is a popular Node.js web framework known for its speed and flexibility. When building APIs, security is paramount. This blog post dives into implementing a simple yet effective way to verify predefined JWT (JSON Web Token) tokens in your Fastify application.
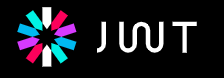
What are JWTs?
Imagine a secure way to identify users across different applications. JWTs are self-contained tokens containing user information and a signature. This signature allows for verifying the token’s authenticity and origin.
Why Use JWT Verification in Fastify?
JWT verification ensures that only authorized users can access specific API endpoints. This is crucial for protecting sensitive data and functionalities within your application.
Step-by-Step: Implementing JWT Verification in Fastify
Here’s a breakdown of the provided code snippet for implementing basic JWT verification in Fastify:
fastify-jwt
package. Install it using your preferred package manager (e.g., npm or yarn) npm install @fastify/jwt
JWT_SECRET
. This secret key is used to sign and verify JWT tokens. Never store this secret directly in your code! Use environment variables or secure configuration management tools.fastify-jwt
: The invoiceRoutes
function registers the fastify-jwt
plugin with the Fastify instance. This plugin provides functionalities for handling JWTs.onRequest
Hook: This hook is triggered for every incoming request. It attempts to verify the JWT token usingrequest.jwtVerify()
. If verification fails (e.g., invalid token or missing token), an error is sent back to the client.- Secured Routes: The code defines two routes:
/upload-invoicedata
(POST): This route expects a JSON request body following a predefined schema (uploadInvoiceDataRequestSchema
). Access to this route likely requires a valid JWT token due to theonRequest
hook
/invoices
(GET): This route retrieves invoice data. Potentially, a valid JWT token might be needed for authorization as well.
invoice.route.ts
import { FastifyInstance } from "fastify"; import { getInvoicesDataHandler, uploadInvoiceDataHandler, } from "./invoice.controller"; import { $INVOICERef } from "./invoice.schema"; import fastifyJwt from "@fastify/jwt"; export async function invoiceRoutes(server: FastifyInstance) { server.register(fastifyJwt, { secret: process.env.JWT_SECRET, }); server.addHook("onRequest", async (request, reply) => { try { await request.jwtVerify(); } catch (err) { reply.send(err); } }); server.post( "/upload-invoicedata", { schema: { body: $INVOICERef("uploadInvoiceDataRequestSchema"), }, }, uploadInvoiceDataHandler, //controller function to handle the actions to executed ); server.get("/invoices", getInvoicesDataHandler); }
How to Generate Predefined JWT Token
To generate predefined tokens, we can do it online through JWT token websites.
- Go to Jwt.io website
- Step 1: Enter your SECRET_KEY instead of sample–KEY
- Step 2: Copy the generated JWT token and share it along with your API
- If you want you can add an optional payload
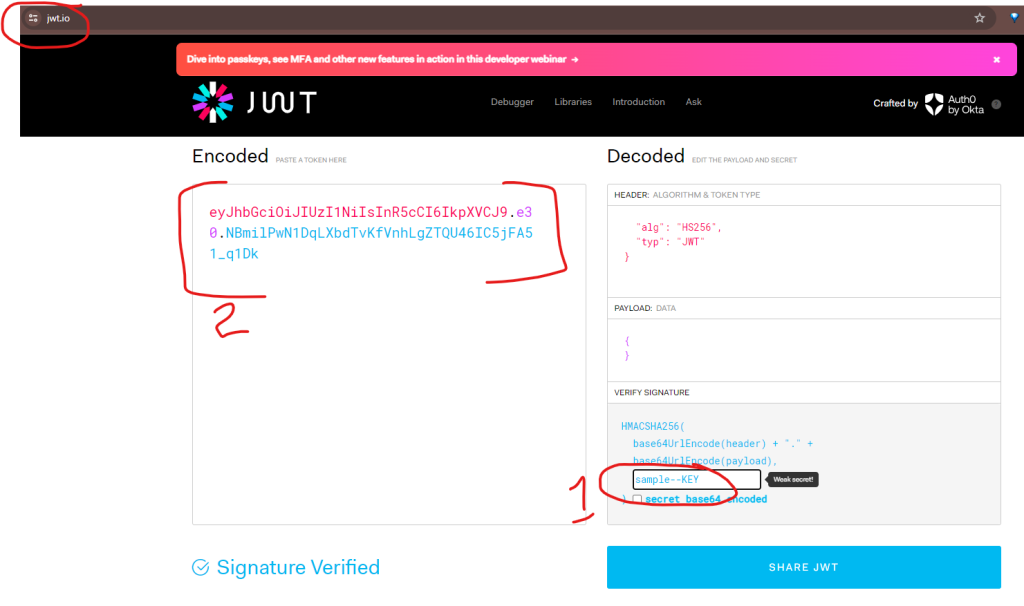
Benefits of this Approach:
- Simple and Effective: The code provides a straightforward way to implement basic JWT verification.
- Improved Security: By verifying tokens, you restrict access to authorized users, protecting sensitive data.
Limitations:
- Predefined Tokens: This approach assumes you have predefined JWT tokens issued elsewhere.
- Custom Logic: For more complex scenarios, you might need to implement custom JWT validation logic.
Next Steps:
This blog post provides a starting point for securing your Fastify application with JWT verification. Consider exploring these next steps:
- Implement custom JWT validation logic for specific scenarios.
- Integrate user authentication and token generation mechanisms.
- Explore advanced security practices for JWT handling.
By following these steps and best practices, you can build robust and secure APIs using Fastify and JWT verification.