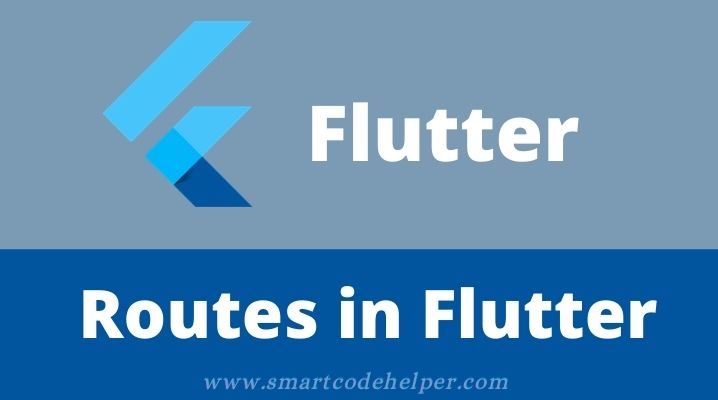
Multiple routes for navigation can be easily handled using routes in flutter. For this we can define list of the routes , set initial route. Then we can use Navigator.pushNamed for specifying the route.
Here is the sample codes:
Defining routes Multiple routes in Flutter
routes: { '/' : (context) => Screen0(), '/first': (context) => Screen1(), '/second': (context) => Screen2(), },
Setting Inital Route in FLutter
initialRoute: '/',
Flutter Routes Sample Codes:
main.dart
import 'package:flutter/material.dart'; import 'package:navigation_demo_starter/screen0.dart'; import 'screen1.dart'; import 'screen2.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( initialRoute: '/', routes: { '/' : (context) => Screen0(), '/first': (context) => Screen1(), '/second': (context) => Screen2(), }, ); } }
screen0.dart
import 'package:flutter/material.dart'; class Screen0 extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( backgroundColor: Colors.purple, title: Text('Screen 0'), ), body: Center( child: Column( children: <Widget>[ RaisedButton( color: Colors.red, child: Text('Go To Screen 1'), onPressed: () { Navigator.pushNamed(context, '/first'); }, ), RaisedButton( color: Colors.blue, child: Text('Go To Screen 2'), onPressed: () { Navigator.pushNamed(context,'/second'); }, ), ], ), ), ); } }
screen1.dart
import 'package:flutter/material.dart'; import 'screen2.dart'; class Screen1 extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( backgroundColor: Colors.red, title: Text('Screen 1'), ), body: Center( child: RaisedButton( color: Colors.red, child: Text('Go Forwards To Screen 2'), onPressed: () { Navigator.push(context, MaterialPageRoute(builder: (context){ return Screen2(); })); }, ), ), ); } }
screen2.dart
import 'package:flutter/material.dart'; class Screen2 extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( backgroundColor: Colors.blue, title: Text('Screen 2'), ), body: Center( child: RaisedButton( color: Colors.blue, child: Text('Go Back To Screen 1'), onPressed: () { Navigator.pop(context); }, ), ), ); } }
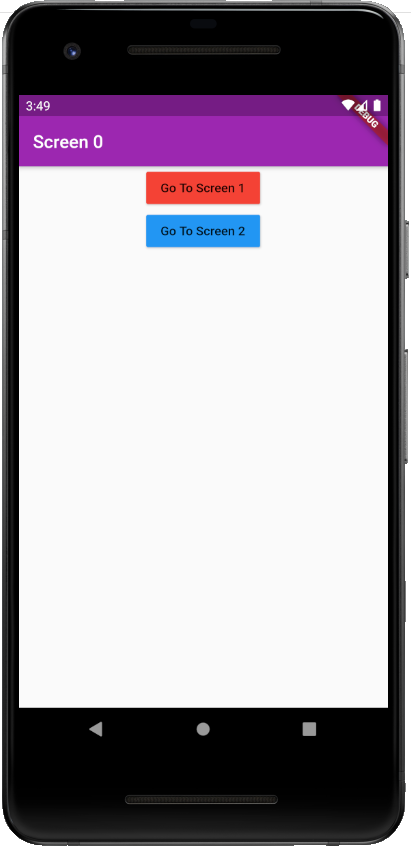
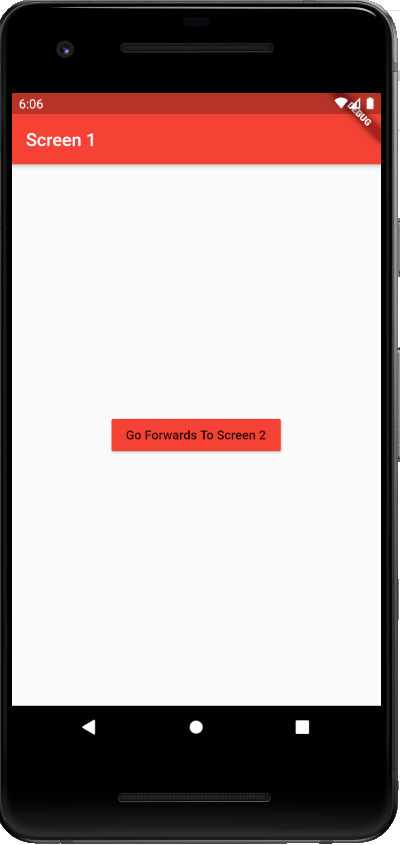
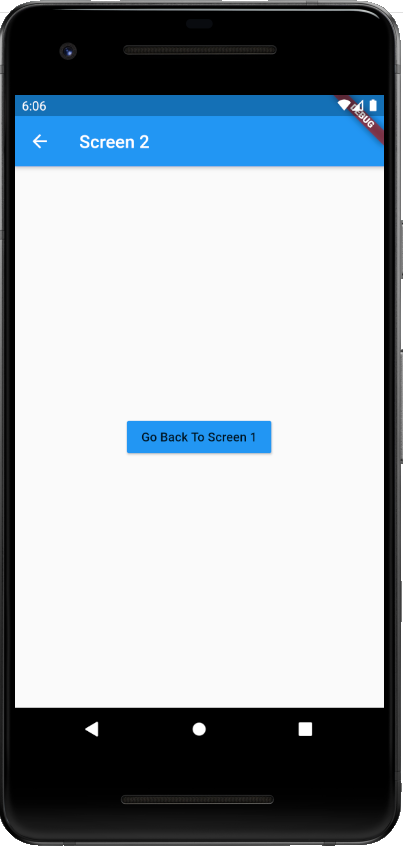