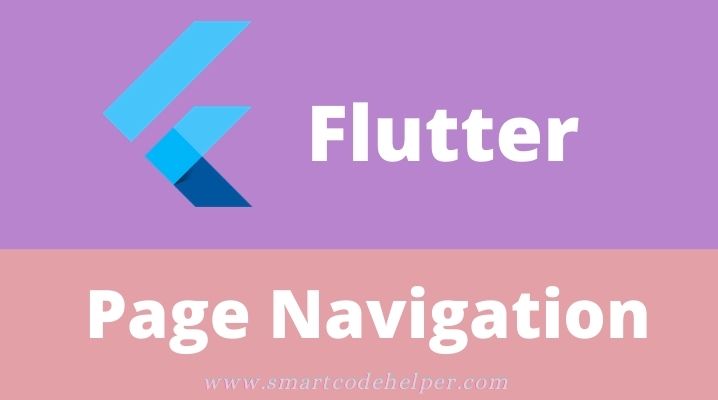
In this post, we gonna check how to navigate from one page to another in flutter. So here we will be moving from screen1 to screen2 on a button click. And back from screen2 to screen one on another button click from the new screen.
Flutter Page Navigation Route – Sample Code
onPressed: () { Navigator.push(context, MaterialPageRoute(builder: (context){ return Screen2(); })); },
screen1.dart
import 'package:flutter/material.dart'; import 'screen2.dart'; class Screen1 extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( backgroundColor: Colors.red, title: Text('Screen 1'), ), body: Center( child: RaisedButton( color: Colors.red, child: Text('Go Forwards To Screen 2'), onPressed: () { Navigator.push(context, MaterialPageRoute(builder: (context){ return Screen2(); })); }, ), ), ); } }
Moving Back in Flutter Navigation
Moving back to old page using navigator pop in flutter navigation and routing.
onPressed: () { Navigator.pop(context); },
screen2.dart
import 'package:flutter/material.dart'; class Screen2 extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( backgroundColor: Colors.blue, title: Text('Screen 2'), ), body: Center( child: RaisedButton( color: Colors.blue, child: Text('Go Back To Screen 1'), onPressed: () { Navigator.pop(context); }, ), ), ); } }
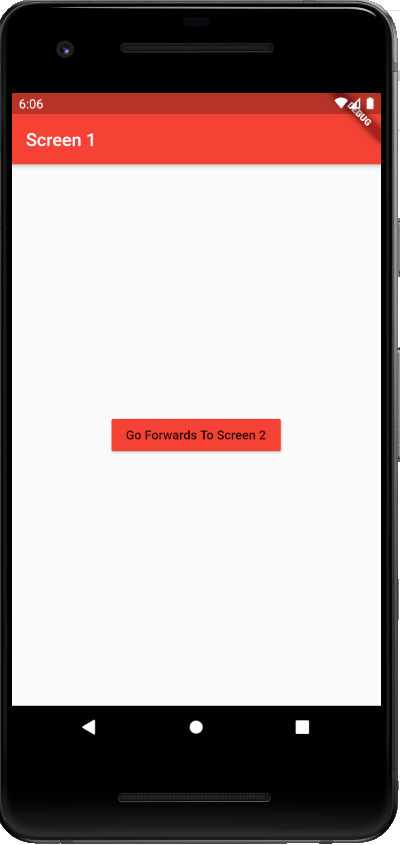
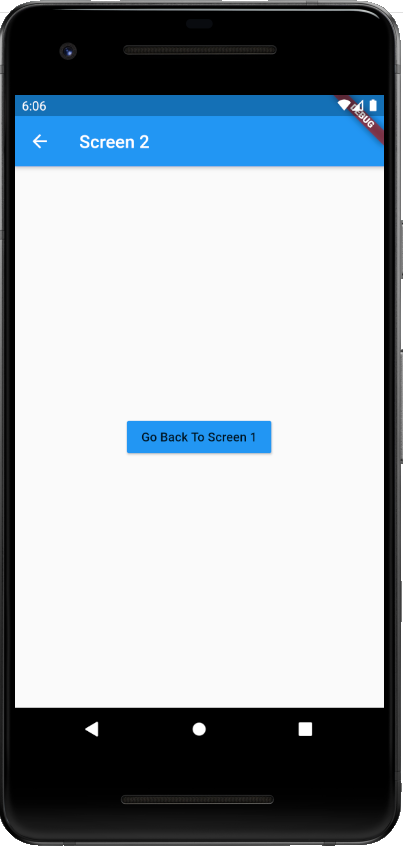