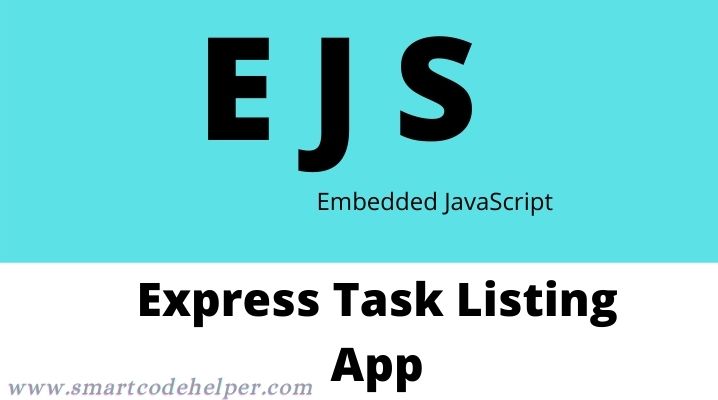
In this post we gonna learn basics of EJS templating for Express JS App. We gonna code a task listing app for the current day. User can add new task to the list from the UI. Then it will be updated to the task list.
In the main page we can see list of tasks. Along with an input field, it can be used by used to add new item to the task list.
Task List App
app.js
const express = require("express"); const https = require("https"); const bodyParser = require("body-parser"); const app = express(); var items = []; app.set('view engine', 'ejs'); app.use(bodyParser.urlencoded({extended:true})); app.post("/", function(req, res){ const item = req.body.input; items.push(item); res.redirect("/"); }); app.get("/", function(req,res){ var today = new Date(); var options = { weekday: "long", day: "numeric", month: "long" }; var day = today.toLocaleDateString("en-US", options); res.render("list", {dayName: day, newListItems: items}); }); app.listen(3000, function(){ console.log("Server started on port 3000"); })
views/list.ejs
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Today's Task List</title> </head> <body> <h1> It's a <%= dayName %>!</h1> <ul> <li> Excercise</li> <li> Cook Food </li> <li> Eat Food</li> <% newListItems.forEach(function(el, index) { %> <li> <%= el %> </li> <% }); %> </ul> <form action="/" method="post"> <label for="input">Add new:</label> <input type="text" name="input" id="input"> <input type="submit" value="Add"> </form> </body> </html>