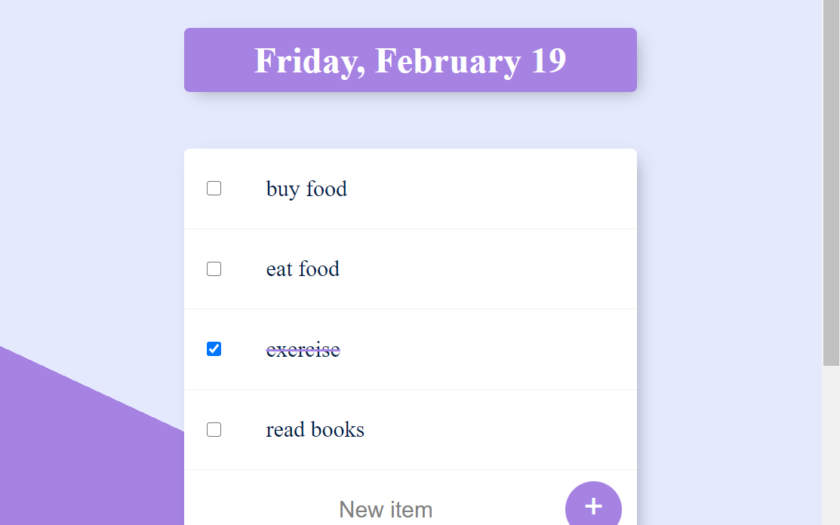
We are creating a sample to-do list app using Node and Express JS. The templating for the app is done using Embedded Javascript (EJS). In this app, we will be creating lists for basic tasks, work tasks as separate pages.
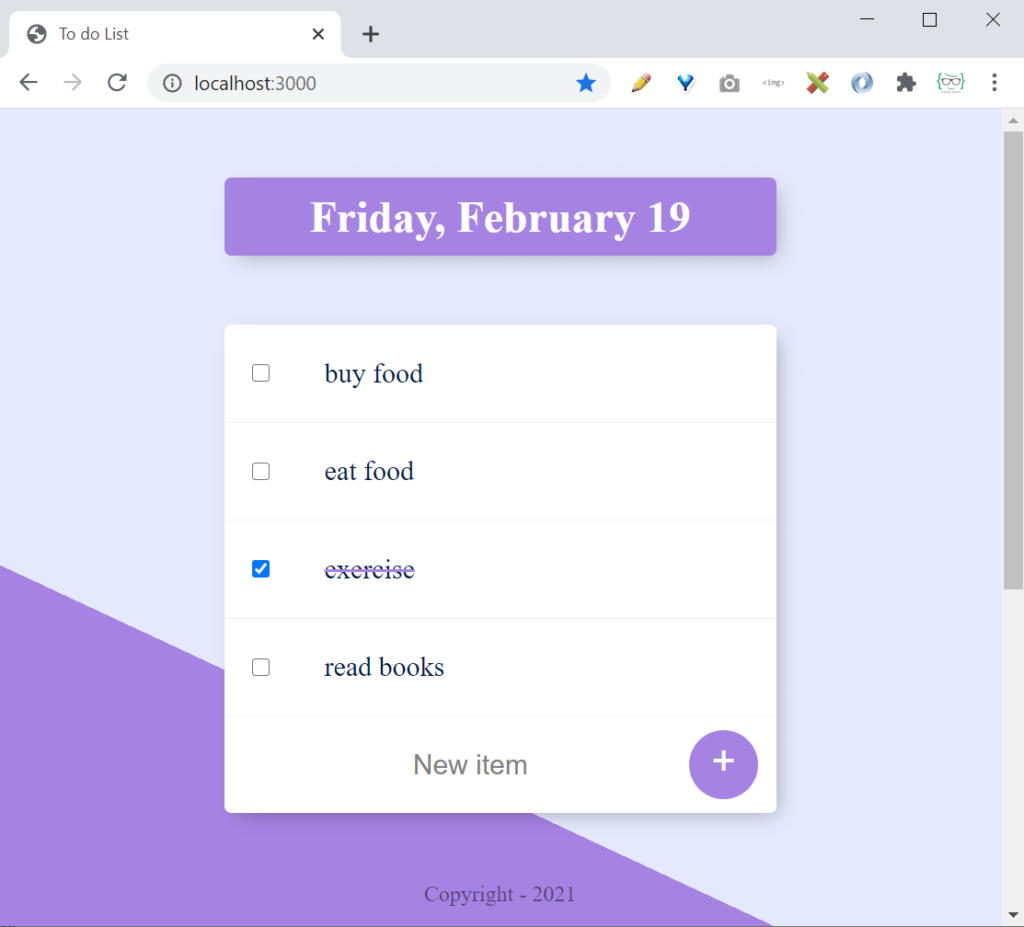
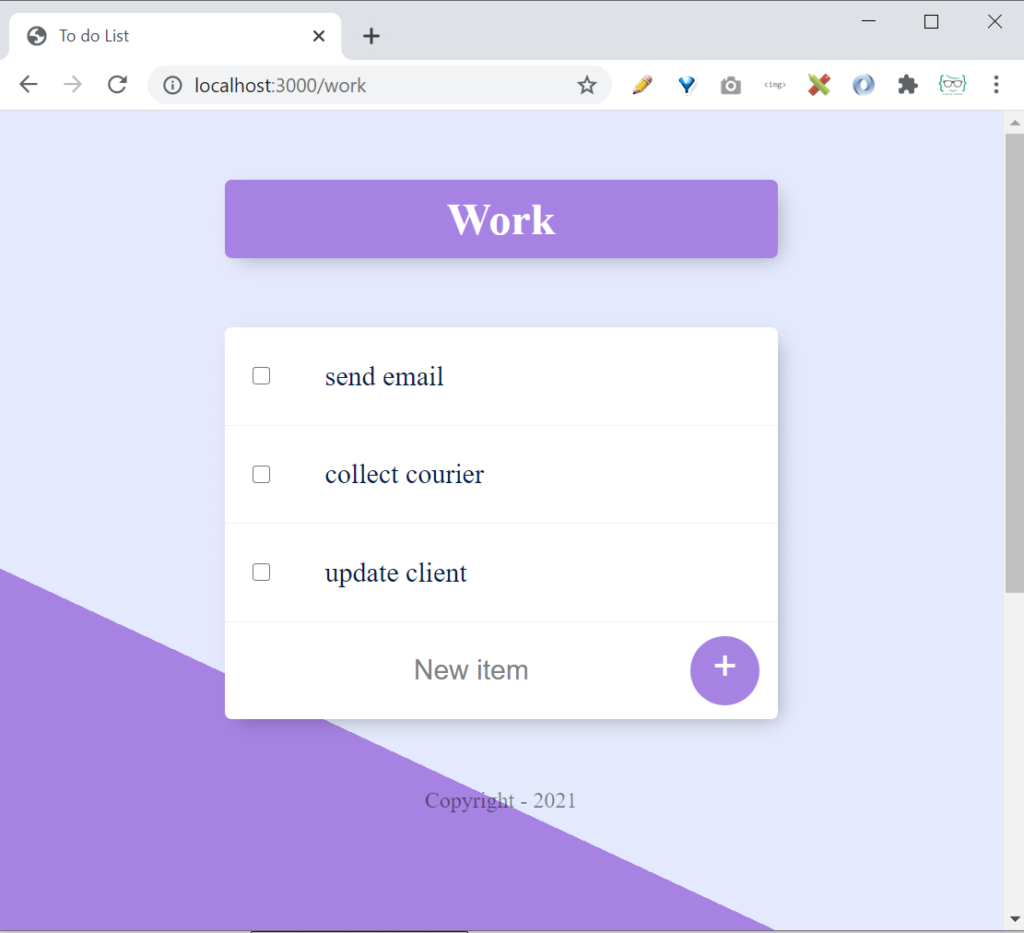
So here is the source code of the to-do list app. The main entry file of the app is app.js.
app.js
const express = require("express"); const bodyParser = require("body-parser"); const date = require(__dirname + "/date.js"); const app = express(); let items = ["buy food", "eat food"]; let workItems = []; app.set('view engine', 'ejs'); app.use(bodyParser.urlencoded({extended:true})); app.use(express.static("public")); app.get("/", function(req,res){ let day = date.getDate(); res.render("list", {listTitle: day, newListItems: items}); }); app.post("/", function(req, res){ let item = req.body.input; //console.log(req.body); if(req.body.submit === 'Work'){ workItems.push(item); res.redirect("/work"); }else{ items.push(item); res.redirect("/"); } }); app.get("/work", function(req,res){ res.render("list", {listTitle:"Work", newListItems: workItems}); }); app.post("/work",function(req, res){ let item = req.body.input; workItems.push(item); res.redirect("/work"); }); app.get("/about", function(req, res){ res.render("about"); }) app.listen(3000, function(){ console.log("Server started on port 3000"); })
date.js
exports.getDate = function(){ const today = new Date(); const options = { weekday: "long", day: "numeric", month: "long" }; return today.toLocaleDateString("en-US", options); } exports.getDay = function() { const today = new Date(); const options = { weekday: "long" }; return today.toLocaleDateString("en-US", options); }
style.css
html { background-color: #E4E9FD; background-image: -webkit-linear-gradient(65deg, #A683E3 50%, #E4E9FD 50%); min-height: 1000px; font-family: 'helvetica neue'; } h1 { color: #fff; padding: 10px; } .box { max-width: 400px; margin: 50px auto; background: white; border-radius: 5px; box-shadow: 5px 5px 15px -5px rgba(0, 0, 0, 0.3); } #heading { background-color: #A683E3; text-align: center; } .item { min-height: 70px; display: flex; align-items: center; border-bottom: 1px solid #F1F1F1; } .item:last-child { border-bottom: 0; } input:checked+p { text-decoration: line-through; text-decoration-color: #A683E3; } input[type="checkbox"] { margin: 20px; } p { margin: 0; padding: 20px; font-size: 20px; font-weight: 200; color: #00204a; } form { text-align: center; margin-left: 20px; } button { min-height: 50px; width: 50px; border-radius: 50%; border-color: transparent; background-color: #A683E3; color: #fff; font-size: 30px; padding-bottom: 6px; border-width: 0; } input[type="text"] { text-align: center; height: 60px; top: 10px; border: none; background: transparent; font-size: 20px; font-weight: 200; width: 313px; } input[type="text"]:focus { outline: none; box-shadow: inset 0 -3px 0 0 #A683E3; } ::placeholder { color: grey; opacity: 1; } footer { color: white; color: rgba(0, 0, 0, 0.5); text-align: center; }
EJS Codes
views/header.ejs
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>To do List</title> <link rel="stylesheet" href="css/styles.css"> </head> <body>
views/footer.ejs
<footer> Copyright - 2021 </footer> </body> </html>
views/list.ejs
<%- include("header") -%> <div class="box" id="heading"> <h1> <%= listTitle %></h1> </div> <div class="box" > <% newListItems.forEach(function(el, index) { %> <div class="item"> <input type="checkbox" > <p> <%= el %> </p> </div> <% }); %> <form class="item" action="/" method="post"> <input type="text" name="input" id="input" placeholder="New item" autocomplete="off"> <button type="submit" id="submit" name="submit" value=<%= listTitle %> >+</button> </form> </div> <%- include("footer") -%>
views/about.ejs
<%- include("header") -%> <h2> About Page</h2> <p> This is the sample about page.</p> <%- include("footer") -%>