How to start with nest js. Install and setup Nest JS framework.
Install nest CLI
npm i -g @nestjs/cli
Go to your folder to create project
nest new project-name
Now it will ask for the package manager choose your fav

Run using yarn
yarn run start:dev
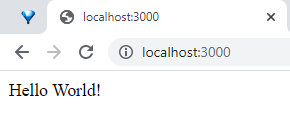
main.ts
is the entry point
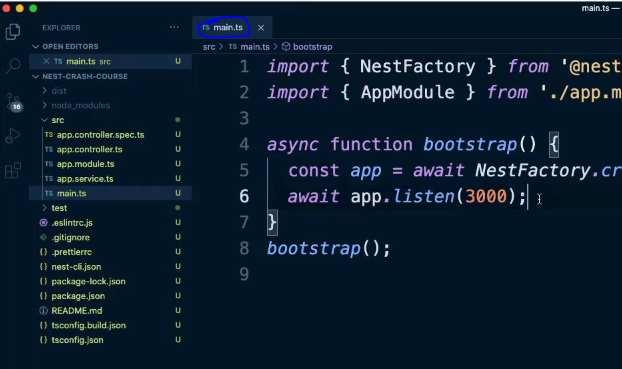
Architecture Nest JS
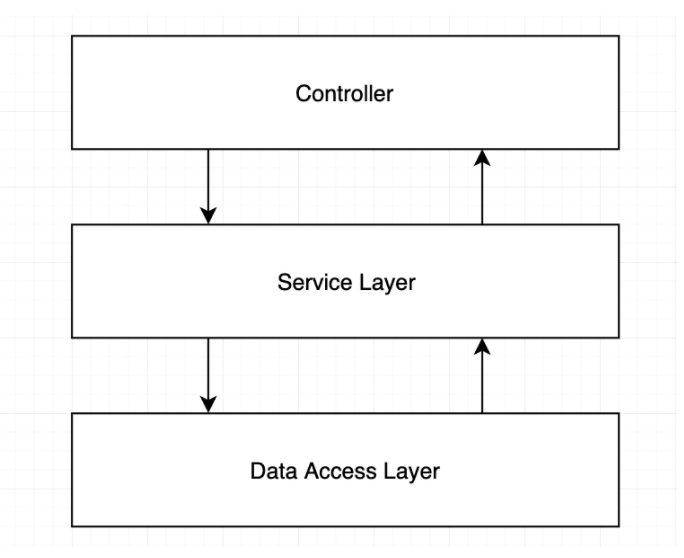
Read More: https://dev.to/santypk4/bulletproof-node-js-project-architecture-4epf
Creating New Module in nest JS
nest generate module users
It will generate new module named users
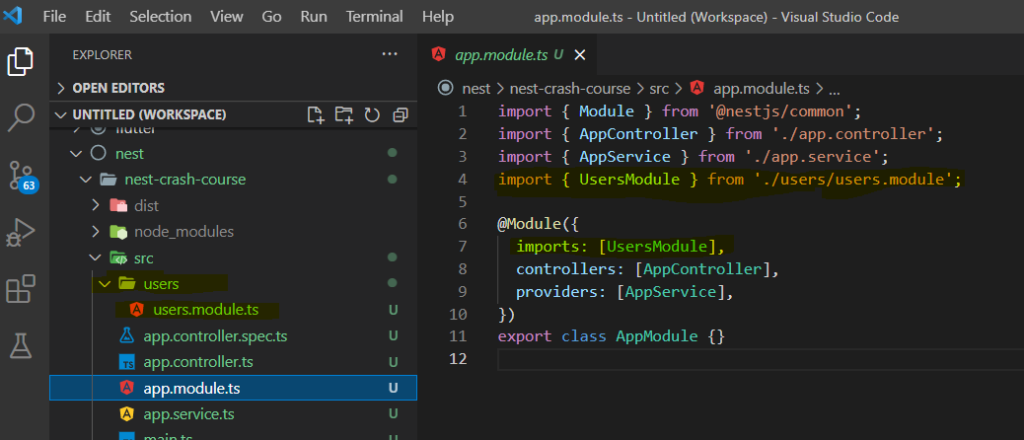
- Generating controllers for users
nest g controller users
- Generating service for users
nest g service users
users.controller.ts
import { Controller, Get } from '@nestjs/common'; @Controller('users') export class UsersController { @Get() getUsers(): any{ return [{id: "101"}]; } }
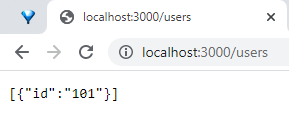
Parsing URL params in Nest JS
import { Controller, Get, Param } from '@nestjs/common'; @Controller('users') export class UsersController { @Get() getUsers(): any{ return [{id: "101"}]; } //parsing url params @Get(':id') getUserById(@Param('id') id: string): any{ return{ id } } }
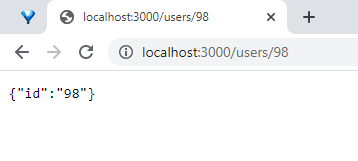
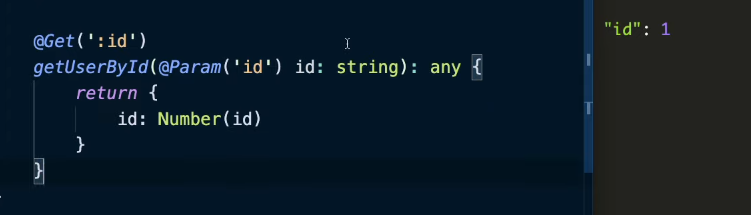