Creating a new Service / Provider in the nest JS app. How to create a Service / Provider in nest JS?
Providers in Nest JS also known as services are basically responsible for executing the business logic. In the Nest JS Service we use @Injectable decorator for the purpose of dependency injection.
Creating a new Service in Nest JS – ( Manual way )
For example, here we are creating a service for the auth functionality of our app.
src
folder create folder named auth auth.service.ts
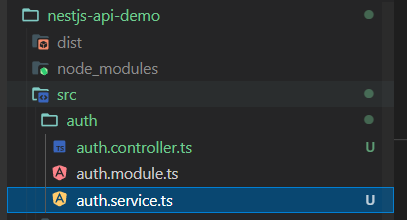
auth.service.ts
import { Injectable } from "@nestjs/common"; @Injectable({}) export class AuthService {}
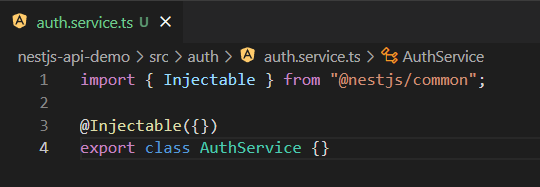
Adding the provider to the module
app.module.ts
import { Module } from '@nestjs/common' import { AuthController } from './auth.controller'; import { AuthService } from './auth.service'; @Module({ controllers: [AuthController], providers: [AuthService] }) export class AuthModule { }
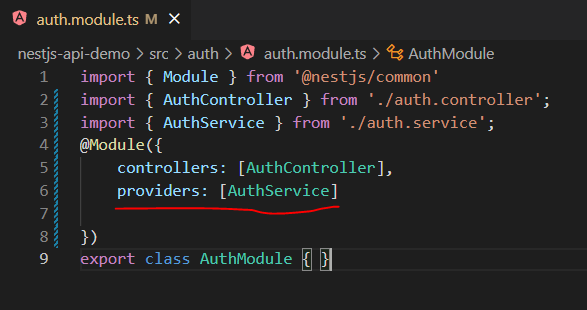
Dependency injection: Connecting Provider to the controller
auth.controller.ts
import { Controller, Post } from "@nestjs/common"; import { AuthService } from "./auth.service"; @Controller('auth') export class AuthController{ constructor(private authService: AuthService) {} @Post('signup') signup() { return this.authService.signup() } @Post('login') login() { return this.authService.login() } }
auth.service.ts
import { Injectable } from "@nestjs/common"; @Injectable({}) export class AuthService { signup(){ return { msg: 'I have signed up!'}; } login(){ return { msg: 'I have logged in..!'}; } }
Result:
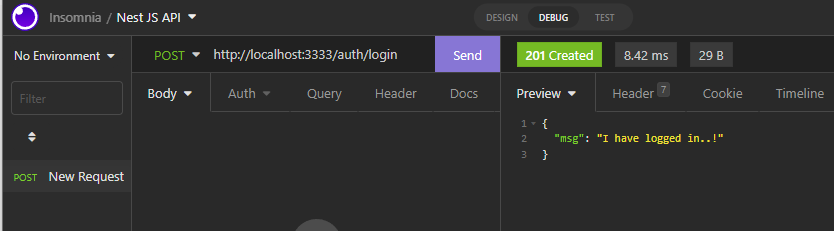
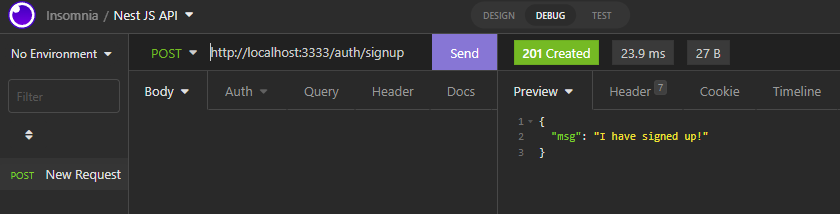