Creating a new module in the nest JS app. How to create a module in nest JS?
Modules basically helps us to break our app into smaller components.
Let’s create an auth module in our newly created nest js app:
Creating a new module in Nest JS – Manual way
- In the
src
folder create folder named auth - Inside the auth folder we can create the module file :
auth.module.ts
import { Module } from '@nestjs/common' @Module({}) export class AuthModule { }
The basic code for a module is given above.
The class is annotated by a @Module()
decorator, which makes it a module.
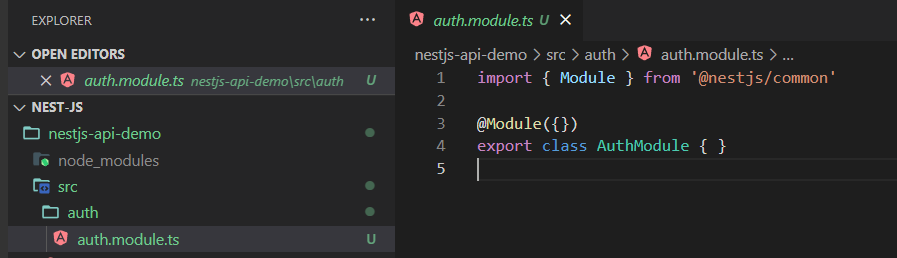
Importing the newly created module inside the app module
import { Module } from '@nestjs/common'; import { AuthModule } from './auth/auth.module'; @Module({ imports: [AuthModule], }) export class AppModule { }
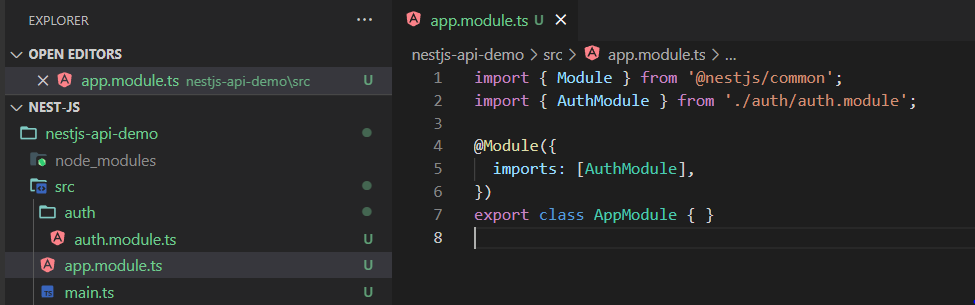
Creating a new module in Nest JS – Using Nest CLI command
Let us see how to create a new module in the nest js app using a CLI command. We are creating a new module for the user called user module using the below command.
nest g module user
g in the above command mean generate
This command will generate our new module for user
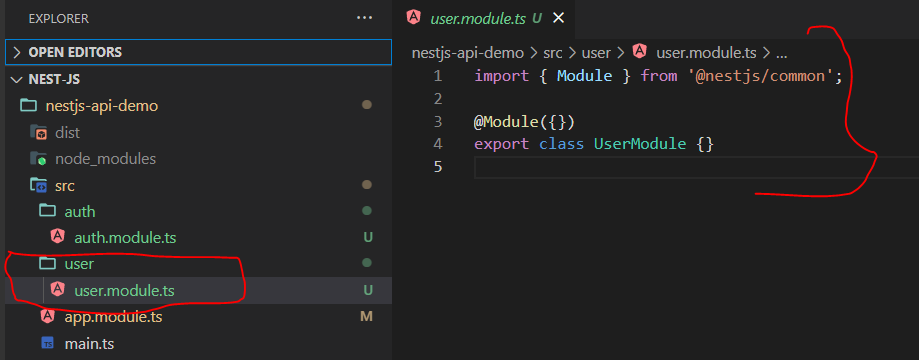
Also, it automatically imports to the App Module
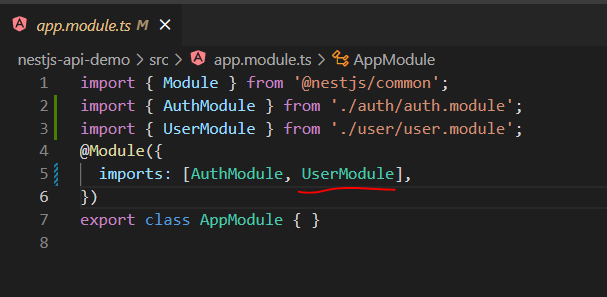
Related: